When working with file operations in VB.NET, reading binary data efficiently is often required. One of the most effective methods for handling binary data in VB.NET is the System.io.file.readallbytes vb.net
method. This method is designed to read all bytes from a specified file and return them as a byte array. Understanding how to utilize this method effectively can simplify file handling in many applications.
Understanding system.io.file.readallbytes vb.net
The system.io.file.readallbytes vb.net
method is part of the System.IO namespace. It allows developers to read the entire content of a file as a byte array. This is particularly useful when working with binary files, such as images, videos, or executable files, where processing raw data is necessary.
Syntax:
Dim fileBytes As Byte() = System.IO.File.ReadAllBytes(filePath)
The method takes a file path as an argument and returns a byte array containing the file’s content.
How system.io.file.readallbytes vb.net Works
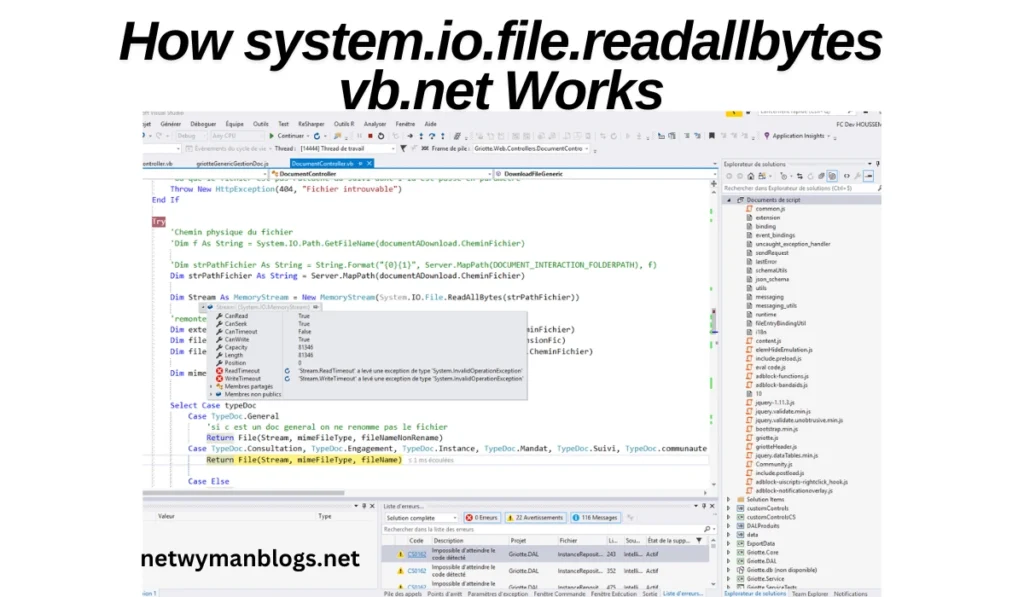
When the system.io.file.readallbytes vb.net
the method is called, it opens the specified file, reads its content into memory as a byte array, and then closes the file. This process ensures that the entire file is read in a single operation, making it efficient for binary data handling.
Example Code
Imports System.IO
Module Module1
Sub Main()
Dim filePath As String = "C:\example\file.jpg"
Try
Dim fileBytes As Byte() = File.ReadAllBytes(filePath)
Console.WriteLine("File read successfully. Total bytes: " & fileBytes.Length)
Catch ex As Exception
Console.WriteLine("Error: " & ex.Message)
End Try
End Sub
End Module
In this example, the system.io.file.readallbytes vb.net
method reads a file and outputs the total number of bytes read. Exception handling ensures that any errors, like missing files or permission issues, are caught and displayed.
Advantages of Using system.io.file.readallbytes vb.net
The system.io.file.readallbytes vb.net
method offers several benefits:
- Efficiency: It reads the entire file in one operation, reducing overhead.
- Simplicity: Requires minimal code to implement.
- Binary Compatibility: Ideal for handling non-text files.
Read more: www creativegaming net
Limitations and Considerations
While powerful, the system.io.file.readallbytes vb.net
method has some limitations:
- Memory Consumption: Large files can consume significant memory.
- Error Handling: Proper exception handling is crucial.
- Access Rights: Requires appropriate file permissions.
Comparing system.io.file.readallbytes vb.net with Other Methods
Feature | ReadAllBytes | StreamReader |
---|---|---|
Data Type | Binary (Byte Array) | Text (String) |
Usage | Binary files (images, videos) | Text files (logs, config) |
Memory Efficiency | Loads entire file into memory | Reads line-by-line |
Error Handling | Requires exception handling | Simpler for text handling |
Best Practices for Using system.io.file.readallbytes vb.net
- File Size Management: Avoid reading very large files; consider streaming for large data.
- Exception Handling: Always use
Try...Catch
to manage errors. - File Path Validation: Ensure the file path is correct and valid.
Common Errors with system.io.file.readallbytes vb.net
- FileNotFoundException: File not found at the specified path.
- IOException: The file is in use by another process.
- UnauthorizedAccessException: Insufficient permissions to access the file.
Example of Error Handling
Try
Dim data As Byte() = File.ReadAllBytes("C:\path\to\file")
Catch ex As FileNotFoundException
Console.WriteLine("File not found.")
Catch ex As UnauthorizedAccessException
Console.WriteLine("Access denied.")
End Try
Real-World Applications of system.io.file.readallbytes vb.net
The system.io.file.readallbytes vb.net
method is widely used in various applications, such as:
- Loading Images: Reading image files for processing or display.
- File Encryption: Reading binary data for encryption or decryption.
- Data Transfer: Sending binary data over a network.
Error Handling
When using System.IO.File.ReadAllBytes
, it’s important to implement error handling to manage potential exceptions, such as FileNotFoundException
, UnauthorizedAccessException
, or IOException
.
Example: Implementing Error Handling
vbCopy codeImports System.IO
Module Module1
Sub Main()
Dim filePath As String = "C:\Files\nonexistentfile.bin"
Try
Dim fileData As Byte() = File.ReadAllBytes(filePath)
Console.WriteLine("File read successfully.")
Catch ex As FileNotFoundException
Console.WriteLine("Error: File not found.")
Catch ex As UnauthorizedAccessException
Console.WriteLine("Error: Access denied.")
Catch ex As IOException
Console.WriteLine("Error: IO exception occurred.")
End Try
End Sub
End Module
By incorporating error handling, your program can gracefully manage exceptions and provide informative messages to the user.
Syntax
vbCopy codeDim fileData As Byte() = File.ReadAllBytes(path)
Here, path
is a string representing the file’s location, and fileData
is the byte array that will hold the file’s contents.
Example Usage:
Suppose you have an image file named “example.jpg” located at “C:\Images”. To read this file into a byte array, you would write:
vbCopy codeImports System.IO
Module Module1
Sub Main()
Dim filePath As String = "C:\Images\example.jpg"
Dim fileData As Byte() = File.ReadAllBytes(filePath)
Console.WriteLine("File read successfully. Number of bytes: " & fileData.Length)
End Sub
End Module
In this example, the File.ReadAllBytes
method reads the entire content of “example.jpg” into the fileData
byte array. The program then outputs the number of bytes read, indicating a successful operation.
Practical Applications of System.IO.File.ReadAllBytes
- Reading Binary Files: This method is ideal for reading binary files such as images, PDFs, or any non-text files into a byte array for processing.
- File Comparison: By reading files into byte arrays, you can compare their contents to determine if they are identical. This is particularly useful in scenarios where file integrity needs to be verified.
Example: Comparing Two Files
To compare two files and check if they are identical, you can use the following function:
vbCopy codeImports System.IO
Module Module1
Function FilesAreEqual(path1 As String, path2 As String) As Boolean
Dim file1 As Byte() = File.ReadAllBytes(path1)
Dim file2 As Byte() = File.ReadAllBytes(path2)
If file1.Length = file2.Length Then
For i As Integer = 0 To file1.Length - 1
If file1(i) <> file2(i) Then Return False
Next
Return True
End If
Return False
End Function
Sub Main()
Dim result As Boolean = FilesAreEqual("C:\Files\file1.bin", "C:\Files\file2.bin")
Console.WriteLine("Files are equal: " & result)
End Sub
End Module
This function reads both files into byte arrays and compares them byte by byte. If all bytes match, the files are considered equal.
Handling Large Files
While System.IO.File.ReadAllBytes
is convenient, it reads the entire file into memory. For very large files, this can lead to memory issues. In such cases, reading the file in smaller chunks using a FileStream
is more efficient.
Example: Reading Large Files in Chunks
vbCopy codeImports System.IO
Module Module1
Sub Main()
Dim filePath As String = "C:\LargeFiles\largefile.bin"
Dim bufferSize As Integer = 1024 ' 1KB buffer
Dim buffer(bufferSize - 1) As Byte
Using fs As New FileStream(filePath, FileMode.Open, FileAccess.Read)
Dim bytesRead As Integer
Do
bytesRead = fs.Read(buffer, 0, bufferSize)
' Process the bytes here
Loop While bytesRead > 0
End Using
End Sub
End Module
This approach reads the file in manageable chunks, reducing memory usage and improving performance when dealing with large files.
Error Handling
When using System.IO.File.ReadAllBytes
, it’s important to implement error handling to manage potential exceptions, such as FileNotFoundException
, UnauthorizedAccessException
, or IOException
.
Example: Implementing Error Handling
vbCopy codeImports System.IO
Module Module1
Sub Main()
Dim filePath As String = "C:\Files\nonexistentfile.bin"
Try
Dim fileData As Byte() = File.ReadAllBytes(filePath)
Console.WriteLine("File read successfully.")
Catch ex As FileNotFoundException
Console.WriteLine("Error: File not found.")
Catch ex As UnauthorizedAccessException
Console.WriteLine("Error: Access denied.")
Catch ex As IOException
Console.WriteLine("Error: IO exception occurred.")
End Try
End Sub
End Module
By incorporating error handling, your program can gracefully manage exceptions and provide informative messages to the user.
Frequently Asked Questions (FAQs)
Can system.io.file.readallbytes vb.net
read large files?
Yes, but it is not recommended for very large files due to high memory usage. Use streaming methods instead.
What happens if the file path is incorrect?
It throws a FileNotFoundException
. Always validate file paths.
Is it suitable for reading text files?
While possible, it is better to use File.ReadAllText
for text files.
How to handle file permission issues?
Use Try...Catch
to manage UnauthorizedAccessException
and ensure proper permissions are set.
Conclusion
The system.io.file.readallbytes vb.net
method is a powerful and efficient tool for reading binary data in VB.NET applications. Its simplicity and effectiveness make it ideal for handling files like images, documents, and executable files. However, developers must be mindful of memory usage and implement proper error handling. By understanding its features, advantages, and limitations, developers can effectively use this method to enhance their applications.